Achieve Cert C++ Compliance Seamlessly with CppDepend
Introduction
In today's competitive software development landscape, maintaining high-quality and secure code is essential. The Cert C++ coding standard serves as a guideline for creating reliable and safe C++ applications. But how can developers efficiently achieve Cert C++ compliance in their projects?
Enter CppDepend, a comprehensive tool specifically designed to help you navigate the complexities of the Cert C++ standard. By utilizing static analysis techniques, CppDepend not only guides you towards compliance, but also empowers you to enhance overall code quality and security.
To learn Cert C++ Standards, you can print this SEI CERT C++ Coding Standard documentation by Aaron Ballman.
Using Cert C++ Rules in CppDepend
When you create a new CppDepend Project, a pop-up will appear displaying all the coding standards you would like to include in your analysis. To include Cert C++ coding standards, check the Cert option and choose Cert C++.
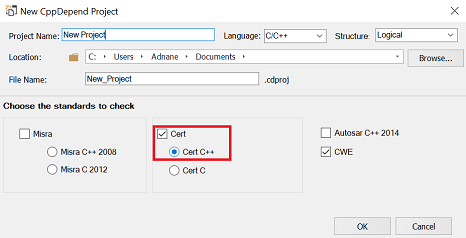
After analyzing your project, Navigate to the Queries and Rules Explorer section, and select the Cert C++ Rules from the left-hand menu (Highlighted in red in the image below). This will display all the related Cert C++ rules on the right. Upon clicking a Rule, the corresponding CQLinq query and the relevant source code will be automatically generated. Additionally, the Metrics View section provides a visualization of the issue's location and significance.
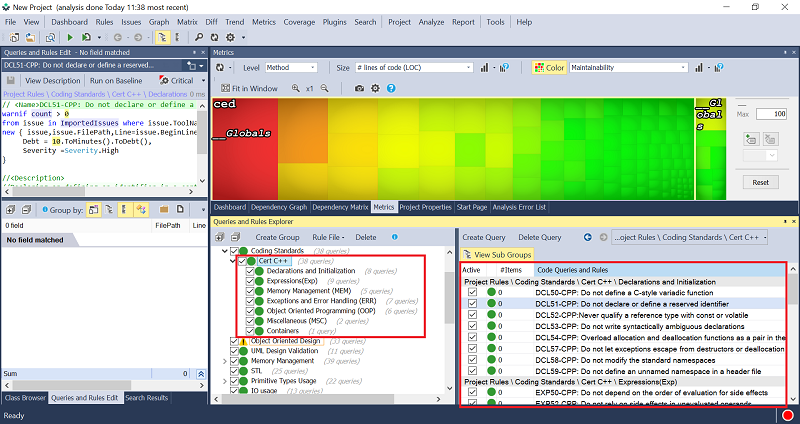
Incorporating Cert C++ Rules into the HTML Report
To include the Cert C++ rules in the generated HTML Report (available in the DevOps Edition), simply right-click on the Cert C++ group and select "List Code Queries of this Group in a dedicated section in Report."
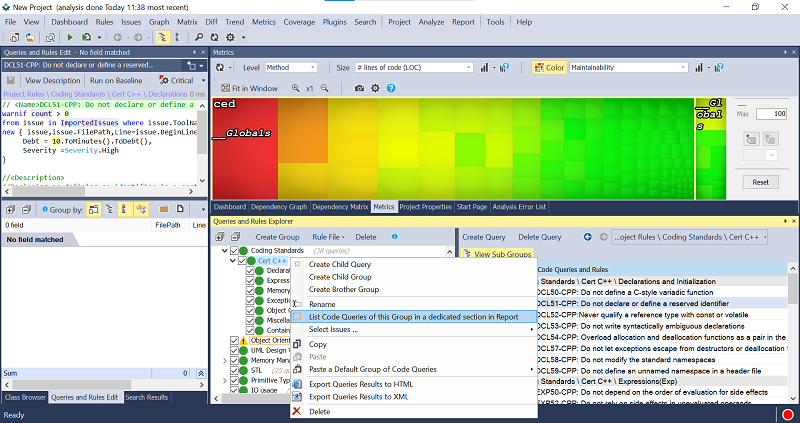
List of Cert C++ Rules
CppDepend offers support for 38 Cert C++ standard queries.To gain a deeper understanding of each rule and its functionality, please consult SEI CERT C++ Coding Standard documentation by Aaron Ballman.
- 8 Declarations and Initialization
- 8 Declarations and Initialization,
- 9 Expressions(Exp),
- 5 Memory Management (MEM),
- 7 Exceptions and Error Handling (ERR),
- 6 Object Oriented Programming (OOP),
- 2 Miscellaneous (MSC),
- 1 Containers
Declarations and Initialization
-
DCL50-CPP: Do not define a C-style variadic function:
Passing arguments via an ellipsis bypasses the type checking performed by the compiler.
Additionally, passing an argument with non-POD class type leads to undefined behaviour.
This coding standard is computed with the following query:
warnif count > 0
from m in JustMyCode.Methods where m.IsVariadic
select m -
DCL51-CPP: Do not declare or define a reserved identifier:
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_pp_macro_is_reserved_id" select
new { issue,issue.FilePath,Line=issue.BeginLine}
-
DCL52-CPP: Never qualify a reference type with const or volatile:
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="err_invalid_reference_qualifier_application" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
DCL53-CPP: Do not write syntactically ambiguous declarations:
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_empty_parens_are_function_decl" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
DCL54-CPP: Overload allocation and deallocation functions as a pair in the same scope:
This coding standard is computed with the following query:
warnif count > 0
from n in JustMyCode.Methods.Where(m=>m.SimpleName=="operatornew") where JustMyCode.Methods.Where(m=>m.SimpleName=="operatordelete").Count()==0
select n -
DCL57-CPP: Do not let exceptions escape from destructors or deallocation functions:
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="exceptThrowInDestructor" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
DCL58-CPP: Do not modify the standard namespaces:
This coding standard is computed with the following query:
warnif count > 0 from codeElement in JustMyCode.CodeElements where codeElement.Parents.Where(t=>t.IsNamespace && t.Name=="std").Count()>0
select codeElement -
DCL59-CPP: Do not define an unnamed namespace in a header file:
This coding standard is computed with the following query:
warnif count>0 from n in Namespaces
where n.Name.StartsWith("anonymous_namespace") && n.Name.Contains(".h")
select n
Expressions (Exp)
-
EXP50-CPP: Do not depend on the order of evaluation for side effects:
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_unsequenced_mod_use" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP52-CPP: Do not rely on side effects in unevaluated operands:
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_side_effects_unevaluated_context" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP53-CPP: Do not read uninitialized memory:
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="CppCheck" && (issue.Type=="uninitvar" || issue.Type=="uninitdata") select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP54-CPP: Do not access an object outside of its lifetime:
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_ret_stack_addr_ref" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP55-CPP: Do not access a cv-qualified object through a cvunqualified type:
This coding standard is computed with the following query:
warnif count > 0
from m in JustMyCode.Methods where
m.IsUsing("Keywords.const_cast".AllowNoMatch()) select m -
EXP57-CPP: Do not cast or delete pointers to incomplete classes:
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_delete_incomplete" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP58-CPP: Pass an object of the correct type to va_start:
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_va_start_type_is_undefined" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP61-CPP: A lambda object must not outlive any of its reference captured objects:
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="returnDanglingLifetime"
&& issue.Description.Contains(("lambda")) select new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP62-CPP: Do not access the bits of an object representation that are not part of the object’s value representation:
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_dyn_class_memaccess" select
new { issue,issue.FilePath,Line=issue.BeginLine}
Memory Management (MEM)
-
MEM50-CPP:Do not access freed memory:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="nullPointer"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
MEM51-CPP:Properly deallocate dynamically allocated resources:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_mismatched_delete_new"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
MEM53-CPP. Explicitly construct and destruct objects when manually managing object lifetime:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="uninitdata"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
MEM56-CPP:Do not store an already-owned pointer value in an unrelated smart pointer:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="doubleFree"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
MEM57-CPP: Avoid using default operator new for over-aligned types:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="cert-mem57-cpp"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Exceptions and Error Handling (ERR)
-
ERR51-CPP: Handle all exceptions:
This coding standard is computed with the following query:
warnif count > 0
from m in JustMyCode.Methods where m.IsEntryPoint
&& m.IsUsing("Keywords.generic_catch".AllowNoMatch()) select m -
ERR52-CPP: Do not use setjmp() or longjmp():
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="cert-err52-cpp"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
ERR53-CPP: Do not reference base classes or class data members in a constructor or destructor function-try-block handler:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang"
&& issue.Type=="warn_cdtor_function_try_handler_mem_expr"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
ERR54-CPP: Catch handlers should order their parameter types from most derived to least derived:
This coding standard is computed with the following query:
// <Name></Name>
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang"
&& issue.Type=="warn_exception_caught_by_earlier_handler"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
ERR58-CPP. Handle all exceptions thrown before main() begins executing:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="cert-err58-cpp"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
ERR60-CPP: Exception objects must be nothrow copynconstructible:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="cert-err60-cpp"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
ERR61-CPP: Catch exceptions by lvalue reference:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="catchExceptionByValue"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Object Oriented Programming (OOP)
-
OOP50-CPP: Do not invoke virtual functions from constructors or destructors:
This coding standard is computed with the following query:
from m in JustMyCode.Methods.Where(m=>(m.IsConstructor || m.IsDestructor)
&& m.MethodsCalled.Where(s=>s.IsVirtual).Count()>0)
select m
-
OOP52-CPP: Do not delete a polymorphic object without a virtual destructor:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_delete_non_virtual_dtor"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
OOP53-CPP: Write constructor member initializers in the canonical order:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_initializer_out_of_order"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
OOP54-CPP: Gracefully handle self-copy assignment:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="operatorEqToSelf"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
OOP57-CPP. Prefer special member functions and overloaded operators to C Standard Library functions:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="cert-oop57-cpp"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
OOP58-CPP: Copy operations must not mutate the source object:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="cert-oop58-cpp"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Miscellaneous (MSC)
-
MSC50-CPP: Do not use std::rand() for generating pseudorandom numbers:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="cert-msc50-cpp"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
MSC51-CPP. Ensure your random number generator is properlyseeded:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="cert-msc51-cpp"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Containers
-
CTR50-CPP: Guarantee that container indices and iterators are within the valid range:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_array_index_exceeds_bounds"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}